Currently hustling to catch up still after a bit, thinking it best to at least document my lab work so that I can at least look back on evidence of progress to keep me motivated toward experimenting with more complicated circuits and code. I'm finding myself having to spend a lot of time looking for resources that help me take grasp the material, here's some stuff I found useful personally:
Arduino Crash Course for Absolute Beginners It really is the same material that we've been covering so I felt comfortable skipping through the series, but the layman terminology gave me a bit of a better lexicon to lean on when "thinking" in Arduino
Flex Sensor Introduction and Use This is helping me think of the ecosystem around physical computation, from measuring range of sensors to the appropriate math toward making appropriate circuit decisions
Arduino Programming Tutorial for Beginners still making my way through this, but it's helping me with the syntax issues I'm having.
Resistor Calculator this could be a resource link in the syllabus.
Lab 3, two different ways
Below are two versions of connecting potentiometer to LED. I was following lab 3 line-by-line just to make sure I'm connecting the work with the words...
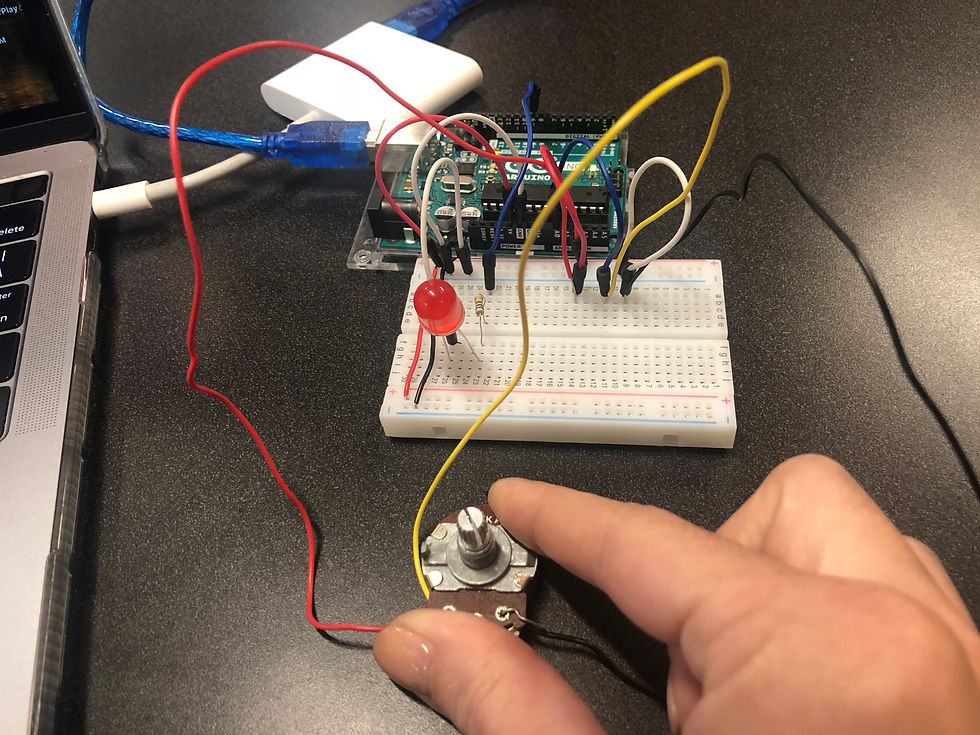
In the first version I had a 10k potentiometer at pin A0, volt in on breadboard #14 and ground at breadboard #9. I Had pin 9 to 560ohm resistor to LED ob breadboard #23, and LED to ground on breadboard #27. Problems here are that the LED faded up to full with potentiometer at halfway, which I addressed in the second attempt, I could use explanation as to which changes caused the smoother fading..
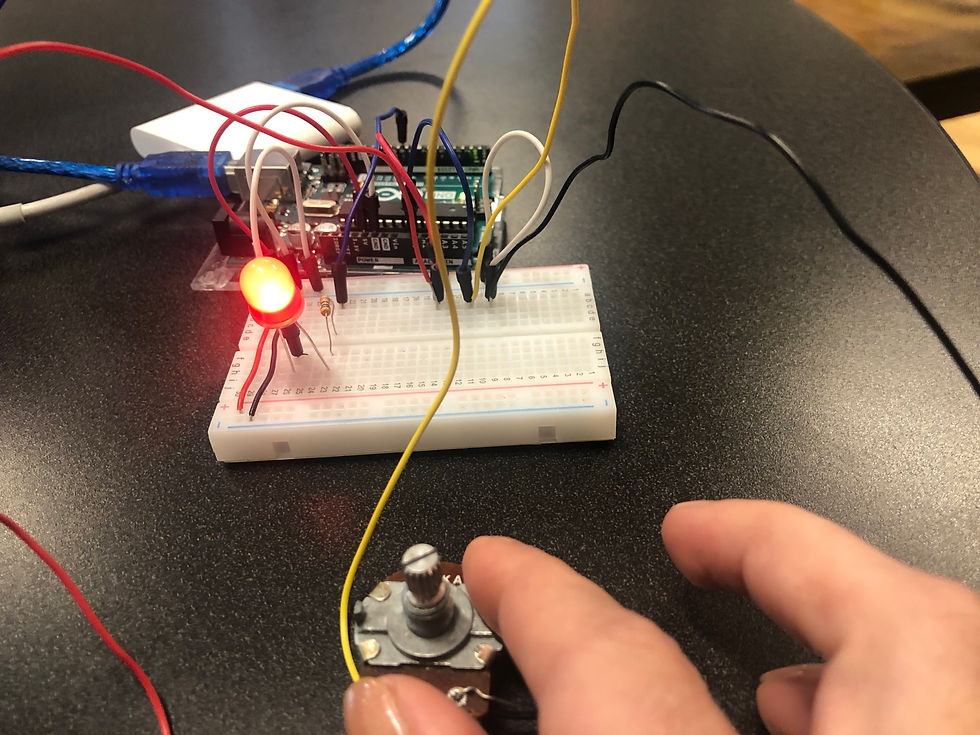
int sensorPin = A0; // select the input pin for the potentiometer
int ledPin = 9; // select the pin for the LED
int brightness = 0;
int analogValue = 0;
int sensorValue = 0;
void setup() {
pinMode(ledPin, OUTPUT);
}
void loop() {
analogValue = analogRead(A0);
brightness = analogValue /4;
analogWrite(ledPin, brightness);
Serial.println(brightness);
delay(sensorValue);
}
On the second attempt, I added a 10k resistor between ground and A0, changed the potentiometer to a 5k, and changed the resistor for the LED to a 220ohm, and used the lab code to map the potentiometer range to the LED range. It led to a smoother brightness fade up/down, and I grasped the sense of mapping ranges.
const int ledPin = 9;
int sensorValue = 0;
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
pinMode(ledPin, OUTPUT);
}
void loop() {
// put your main code here, to run repeatedly:
sensorValue = analogRead(A0);
int brightness = map(sensorValue, 0, 1023, 0, 255);
analogWrite(ledPin, brightness);
Serial.println(sensorValue);
Serial.println(brightness);
}
Lab 4, trash is my treasure
Having the break to really follow the labs line by line has been huge. The vocab is coming into focus, I'm finally getting a basic understanding of how to use the serial monitor to read/measure different figures, and I've been trying to translate the lab code backwards into pseudocode which is helping me develop syntax literacy. Slowly.
Following lab 4 I chose a flex sensor to control the servo, following through using the serial monitor to figure out the range of the flex monitor, which read around 150 when completely flexed, ~290 when relaxed, and ~350 when slightly hyper-flexed. I got a little lost in trying to figure out the math required to determine a resistor value for the circuit, and don't know how to calculate off the serial monitor yet. I went through the forums instead and accordingly went with the highest value < 100k resistor I had (10kohms in this case, which I think was too weak but I'm not sure how it's affecting my circuit technically).
Setup as follows: 5 Volt in to flex sensor through a 10k resistor to ground, pin A0 with cable between flex sensor and resistor/ground. 5 volt in to servo to ground, pin 3. The motor was a little "noisy" when the flex sensor was "relaxed" which could be because of the low resistance value.. I was recommended using a filtering capacitor between voltage and ground, which seemed to help the chatter... But the real lesson of lab 4 was finding the delight...
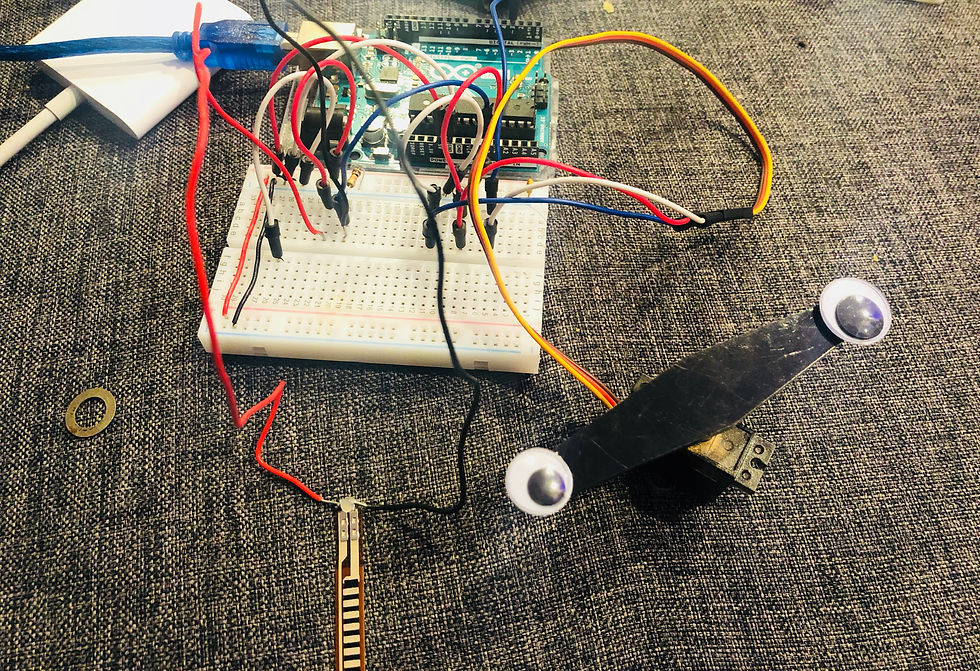
This is a scrap shelf gremlin. I found them while digging through the junked goods looking for breakaway sensor headers for the flex sensor (solved with some tedious wire wrapping and a touch of hot glue). Scrap shelf gremlins come in any shape or size you can imagine, this is the one that came out of my imagination while scavenging. It got me thinking that maybe there's a scrap shelf adopt-a-thon in the future to get people digging in... that place is an absolutely underrated treasure trove. On a personal note, realizing a little anthropomorphism makes this entire class more approachable :)
#include <Servo.h>
Servo servoMotor;
int servoPin = 3;
void setup() {
Serial.begin(9600);
servoMotor.attach(servoPin);
}
void loop() {
// read the input on analog pin 0:
int analogValue = analogRead(A0);
// print out the value you read:
Serial.println(analogValue);
int servoAngle = map(analogValue, 160, 280, 0, 179);
servoMotor.write(servoAngle);
}
pt. 2 following with questions..
Comments